반응형
C#에서 텍스트 파일을 읽고 저장하는 다양한 방법이 있습니다. 일반적으로 System.IO 네임스페이스를 사용하여 파일을 처리할 수 있습니다. 아래 몇 가지 방법을 설명하겠습니다.
1. File.ReadAllText 및 File.WriteAllText
텍스트 파일의 모든 내용을 한 번에 읽거나 저장할 때 사용합니다.
using System.IO; // 파일 읽기 string content = File.ReadAllText("파일경로.txt"); Console.WriteLine(content); // 파일 저장 File.WriteAllText("파일경로.txt", "저장할 내용"); |
예제
using System;
//using System.Collections.Generic;
//using System.Linq;
//using System.Text;
//using System.Threading.Tasks;
using System.IO;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string path = "test-1.txt";
string contents = "C#에서 텍스트 파일을 읽고 저장하는\r\n다양한 방법이 있습니다.";
// 파일 저장
File.WriteAllText(path, contents);
// 파일 읽기
string content = File.ReadAllText(path);
Console.WriteLine(content);
}
}
}
2. File.ReadAllLines 및 File.WriteAllLines
파일을 줄 단위로 읽고, 줄 단위로 저장할 때 사용합니다.
using System;
using System.IO;
namespace ConsoleApp1
{
internal class Program
{
static void Main(string[] args)
{
string path = "test-1.txt";
// 파일 저장 (줄 단위)
string[] newLines = { "첫 줄", "두 번째 줄", "세 번째 줄" };
File.WriteAllLines(path, newLines);
// 파일 읽기 (줄 단위)
string[] lines = File.ReadAllLines(path);
foreach (string line in lines)
{
Console.WriteLine(line);
}
}
}
}
3. FileStream 및 StreamWriter, StreamReader 사용
파일을 더 세밀하게 제어할 수 있는 방법입니다. 특히 대용량 파일을 처리하거나 파일을 부분적으로 읽고 쓸 때 유용합니다.
StreamReader: 파일 읽기
using System.IO;
// StreamReader로 파일 읽기
using (StreamReader reader = new StreamReader("파일경로.txt"))
{
string line;
while ((line = reader.ReadLine()) != null)
{
Console.WriteLine(line);
}
}
StreamWriter: 파일 저장
using System.IO;
// 텍스트 덧붙이기
File.AppendAllText("파일경로.txt", "추가할 내용\n");
// StreamWriter로 덧붙이기
using (StreamWriter writer = new StreamWriter("파일경로.txt", true))
{
writer.WriteLine("추가할 줄");
}
이 방법들은 파일 크기와 사용 목적에 따라 선택할 수 있습니다. 파일이 작다면 ReadAllText와 WriteAllText가 간편하지만, 대용량 파일에서는 StreamReader와 StreamWriter가 더 효율적일 수 있습니다.
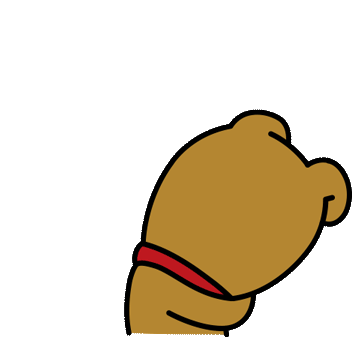
반응형
'C#' 카테고리의 다른 글
데이터베이스 연결 문자열 모음 Database connection string (0) | 2024.10.30 |
---|---|
C#, using 키워드의 3가지 용도 (0) | 2024.10.13 |
(C#) 폼 이벤트: Form_Load, FormClosing, FormClosed, 폼 닫기 (0) | 2024.05.14 |
(C#) MDI 다중 문서 인터페이스 Multiple Document Interface (0) | 2023.11.30 |
(C#) ComboBox, Dictionary(key, value) 사용하기 (0) | 2023.11.09 |